OAuth Client Credentials
The Proof API supports the use of the client_credentials
grant type as an alternative form of authentication, as defined under the OAuth 2.0 specification.
Provisioning a new client application
- Log in to app.proof.com as an Admin or Owner user.
- Navigate to Settings in the left navigation bar and select the OAuth settings menu.
- Select "Create client application" and provide a unique display name for the client app - this display name can be edited at any time.
-
Take note of your client secret
While you can view the app ID at any time, the client secret is only available to view once. You will need to provision a new secret if you lose it, so store it somewhere safe!
You can provision as many client secrets as needed for a given client application.
Request an access token
Initiate a POST
call to https://api.proof.com/oauth/v2/token
(for Production) or https://api.fairfax.proof.com/oauth/v2/token
(for Fairfax/sandbox). Provide your client_id
and client_secret
through a Basic auth header.
You may receive a 415
HTTP status response if Content-Type: application/x-www-form-urlencoded
is not passed in the request headers.
import requests
url = "https://api.fairfax.proof.com/oauth/v2/token"
client_id = "your_client_id"
client_secret = "your_client_secret"
response = requests.post(
url,
data={
"grant_type": "client_credentials"
},
auth=(client_id, client_secret)
)
token = response.json().get("access_token")
{
"access_token":"your_token",
"token_type":"Bearer",
"expires_in":7200,
"scope":"read write",
"created_at":1746822886
}
In an API client like Postman, this could look like so:
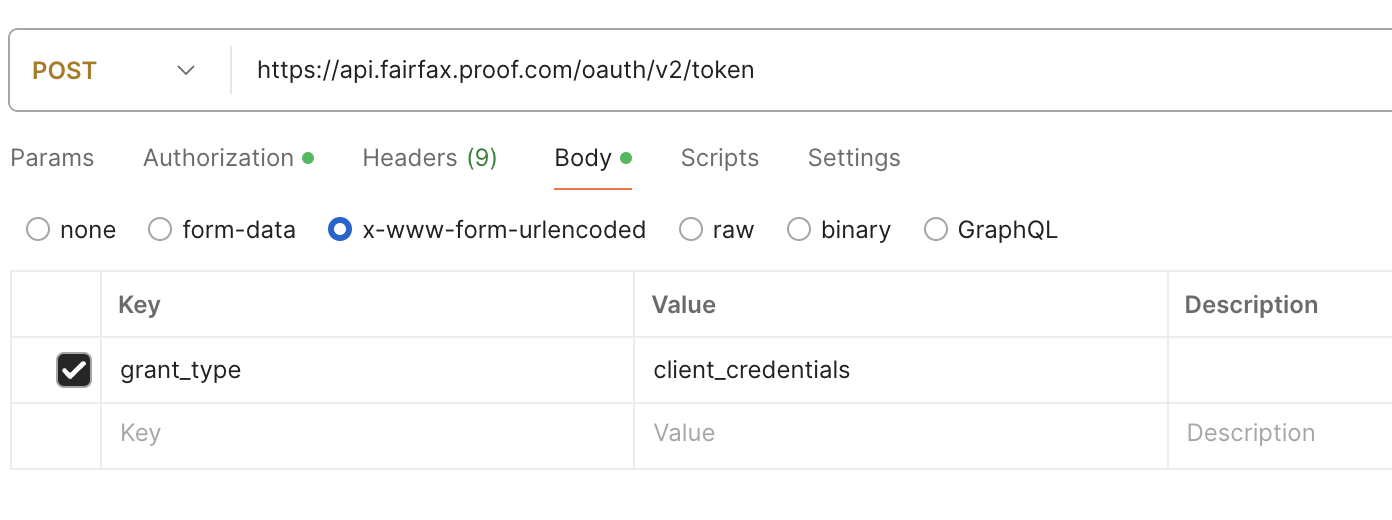
In the example below the obfuscated values starting with ca
and 4a5f
represent the client_id
and client_secret
in the basic auth header.
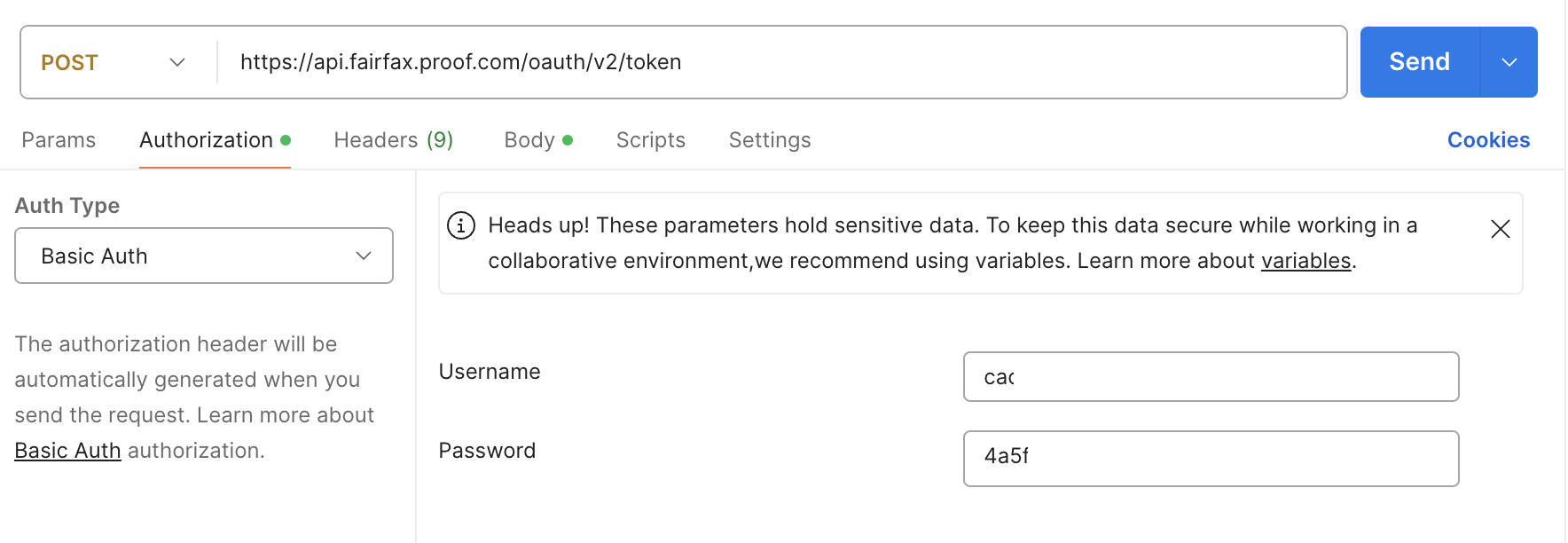
Check status of an existing token
You can check the token expiry, application ID, and token creation timestamp at any time by making a GET
call to api.proof.com/oauth/v2/token/info
, providing the token through a Bearer auth header.
url = "https://api.fairfax.proof.com/oauth/v2/token/info"
token = "your_access_token"
headers = {
"Authorization": f"Bearer {token}"
}
response = requests.get(url, headers=headers)
print(response.status_code)
{
"resource_owner_id":null,
"scope":["read","write"],
"expires_in":5179,
"application":{
"uid":"your_app_id"
},
"created_at":1746822886
}
Authenticate using the token
Initiate a call to any Proof endpoint. Rather than providing an ApiKey
header, provide your token through a Bearer auth header.
url = "https://api.fairfax.proof.com/v1/transactions/otxxxxxxx"
token = "your_access_token"
headers = {
"Authorization": f"Bearer {token}"
}
response = requests.get(url, headers=headers)
print(response.text)
Updated about 1 month ago